Docker has a host of instruction commands. These are commands that are put in the Docker File. Let’s look at the ones which are available.
CMD Instruction
This command is used to execute a command at runtime when the container is executed.
Syntax
CMD command param1
Options
- command − This is the command to run when the container is launched.
- param1 − This is the parameter entered to the command.
Return Value
The command will execute accordingly.
Example
In our example, we will enter a simple Hello World echo in our Docker File and create an image and launch a container from it.
Step 1 − Build the Docker File with the following commands −
FROM ubuntu MAINTAINER demousr@gmail.com CMD [“echo” , “hello world”]
Here, the CMD is just used to print hello world.

Step 2 − Build the image using the Docker build command.
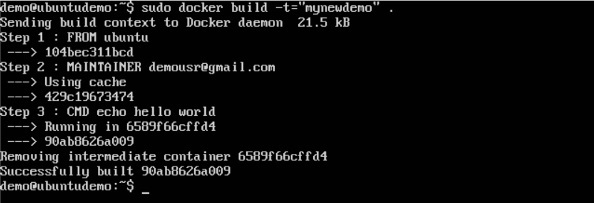
Step 3 − Run a container from the image.

ENTRYPOINT
This command can also be used to execute commands at runtime for the container. But we can be more flexible with the ENTRYPOINT command.
Syntax
ENTRYPOINT command param1
Options
- command − This is the command to run when the container is launched.
- param1 − This is the parameter entered into the command.
Return Value
The command will execute accordingly.
Example
Let’s take a look at an example to understand more about ENTRYPOINT. In our example, we will enter a simple echo command in our Docker File and create an image and launch a container from it.
Step 1 − Build the Docker File with the following commands −
FROM ubuntu MAINTAINER demousr@gmail.com ENTRYPOINT [“echo”]

Step 2 − Build the image using the Docker build command.
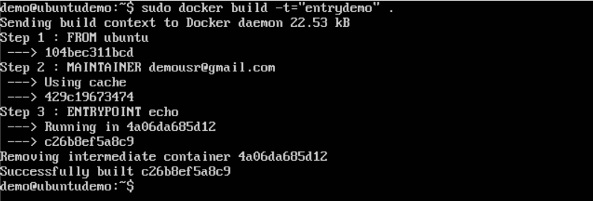
Step 3 − Run a container from the image.
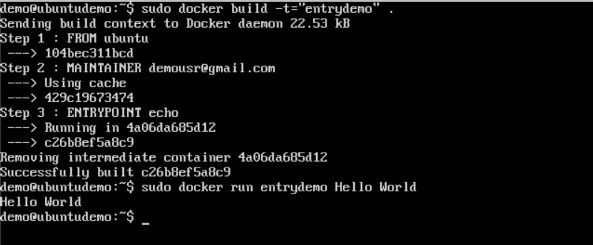
ENV
This command is used to set environment variables in the container.
Syntax
ENV key value
Options
- Key − This is the key for the environment variable.
- value − This is the value for the environment variable.
Return Value
The command will execute accordingly.
Example
In our example, we will enter a simple echo command in our Docker File and create an image and launch a container from it.
Step 1 − Build the Docker File with the following commands −
FROM ubuntu MAINTAINER demousr@gmail.com ENV var1=Tutorial var2=point

Step 2 − Build the image using the Docker build command.
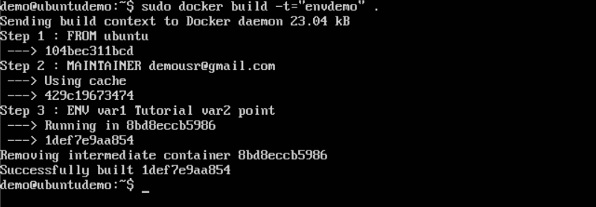
Step 3 − Run a container from the image.
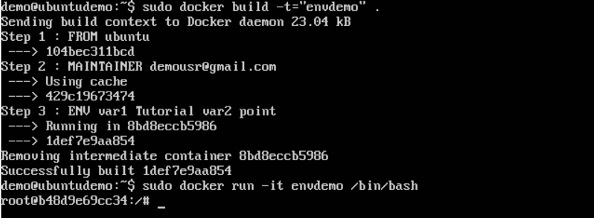
Step 4 − Finally, execute the env command to see the environment variables.
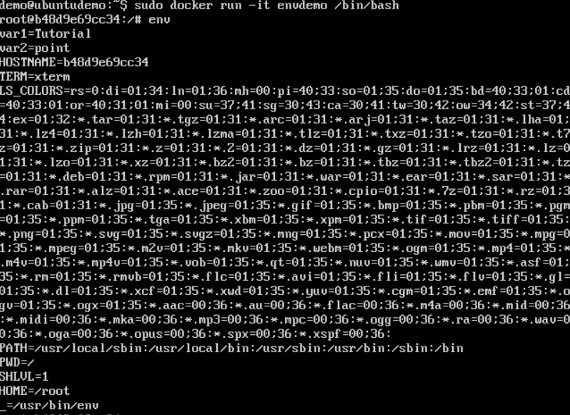
WORKDIR
This command is used to set the working directory of the container.
Syntax
WORKDIR dirname
Options
- dirname − The new working directory. If the directory does not exist, it will be added.
Return Value
The command will execute accordingly.
Example
In our example, we will enter a simple echo command in our Docker File and create an image and launch a container from it.
Step 1 − Build the Docker File with the following commands −
FROM ubuntu MAINTAINER demousr@gmail.com WORKDIR /newtemp CMD pwd
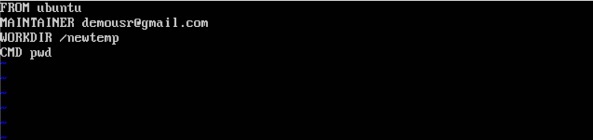
Step 2 − Build the image using the Docker build command.
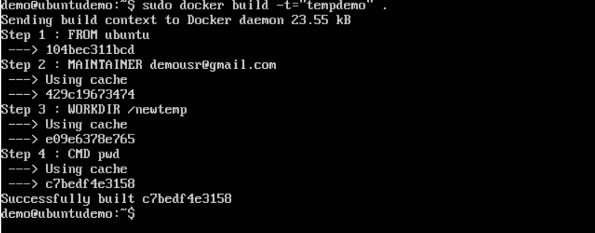
Step 3 − Run a container from the image.
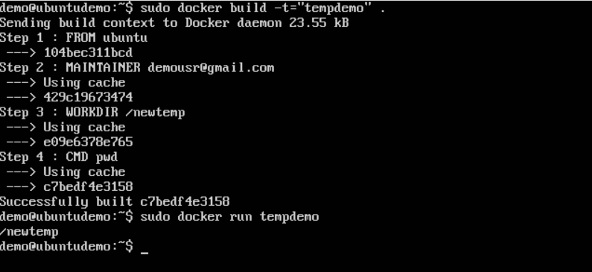
No comments:
Post a Comment