How to Insert, Update and Delete the data available in JSON File in Android ?
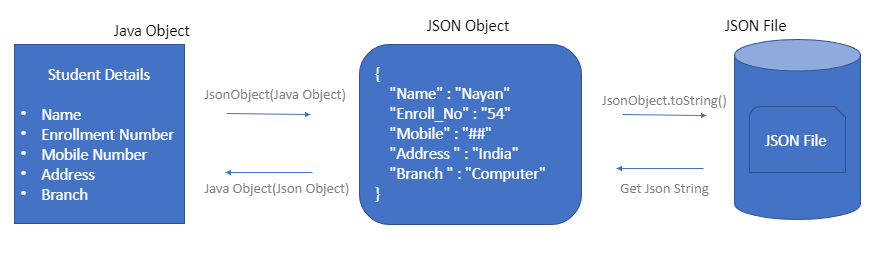
Android provides several ways of dealing with app data within the system or local storage. We are going to be dealing with app-specific storage of data in directory available in Internal or External Storage of system.
App-specific Storage
- Internal Storage : Sensitive data, No other application access it.
- External Storage : Other application can access it like Images.
What we are going to do ?
We will generate a JSON file, which will be stored in Internal storage of application. From android application user will add(WRITE) data, which will be converted into JSON format(JSON Object) and then stored in JSON file.
We will access(READ) the data from JSON file and converted into app usable format like string, arrays etc.
We will also UPDATE the data from JSON file and save it back to JSON file.
We will also perform the DELETION operation on JSON file data/Objects.
- Write Data into JSON File :-
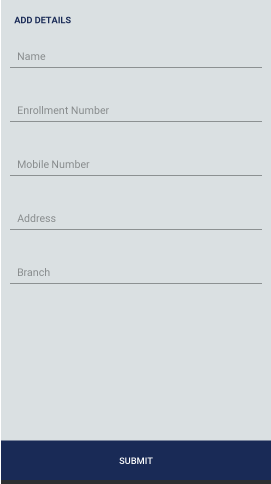
Data will be taken in terms of Java Object and transferred to JSON File.
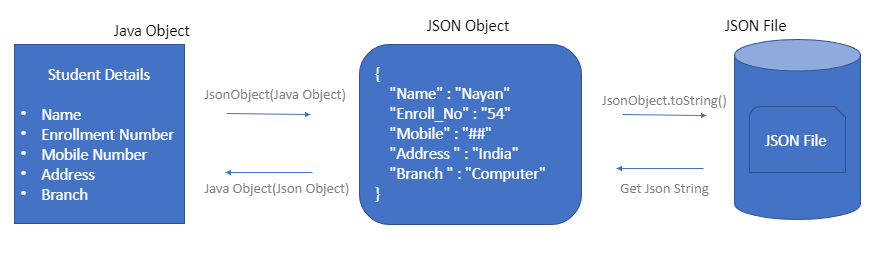
Java-object will be passed to JsonObject, which will convert the java object into JsonObject which means the value is now associated with a key because JSON works as Key-Value pairs.
JSONObject jsonObject = new JSONObject();
jsonObject.put("Name", Name);
jsonObject.put("Enroll_No", Enrollment Number);
jsonObject.put("Mobile", Mobile);
jsonObject.put("Address", Address);
jsonObject.put("Branch", Branch);
return jsonObject;
Now we will store this JsonObject to our JSON File available at Internal Storage, For this we need to define the path and then we will store the JSON object as a String into .Json file.
// Convert JsonObject to String Format
String userString = JsonObject.toString();// Define the File Path and its Name
File file = new File(context.getFilesDir(),FILE_NAME);
FileWriter fileWriter = new FileWriter(file);
BufferedWriter bufferedWriter = new BufferedWriter(fileWriter);
bufferedWriter.write(userString);
bufferedWriter.close();
At this point, Data has entered into JSON file. How can I see where the data is transferred into Android Studio → Device File Explorer.
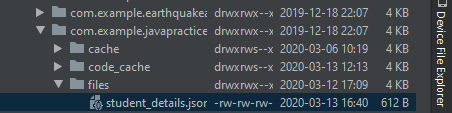
JSON File will have data stored Like this :-
{
"Name":"Ram Varma",
"Enroll_no":"160760120546",
"Mobile":"8989898989",
"Address":"Sahyog Socirty",
"Branch":"EC"
}
2. Read Data From JSON FIle :-
Now we have to access the data which is available in JSON file.
File file = new File(context.getFilesDir(),FILE_NAME);FileReader fileReader = new FileReader(file);
BufferedReader bufferedReader = new BufferedReader(fileReader);
StringBuilder stringBuilder = new StringBuilder();
String line = bufferedReader.readLine();
while (line != null){
stringBuilder.append(line).append("\n");
line = bufferedReader.readLine();
}
bufferedReader.close();// This responce will have Json Format String
String responce = stringBuilder.toString();
This responce is available in String Json Format,but we have to access it in Java Object form so that we can apply it wherever we want in our application.
So we have to get the data available in responce(String) using Key and assign those value to our Java Object.
JSONObject jsonObject = new JSONObject(responce);//Java Object
JavaObject javaObject =
new JavaObject(jsonObject.get("name").toString(),
jsonObject.get("enroll_no").toString(),
jsonObject.get("mobile").toString(),
jsonObject.get("address").toString(),
jsonObject.get("branch").toString());return javaObject;
Now We can access this javaObject and have value which was stored in JSON file.
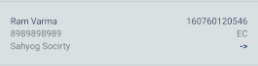
3. Update and Delete the Data to JSON FIle :-
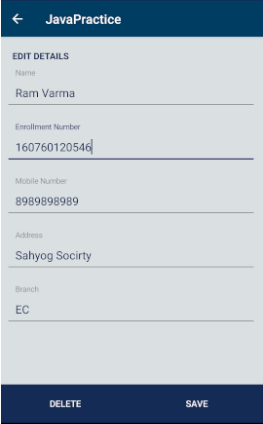
Now This updated Java Object again perform WRITE operation(repeat step 1) on JSON file and Edited data will be displayed when we read(repeat step 2) the JSON object from file.
DELETE will remove the current object and perform the step 1 and step 2 procedure.
Now we can display the data whichever way we want …
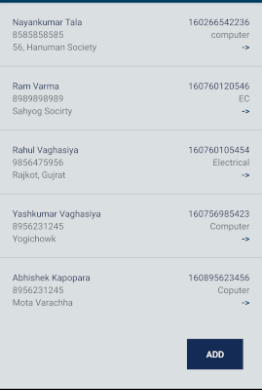
No comments:
Post a Comment