Go has good support for url parsing. URL contains a scheme, authentication info, host, port, path, query params, and query fragment. we can parse URL and deduce what are the parameter is coming to the server and then process the request accordingly.
The net/url package has the required functions like Scheme, User, Host, Path, RawQuery etc.
Go URL Parsing Example 1
- package main
- import "fmt"
- import "net"
- import "net/url"
- func main() {
-
- p := fmt.Println
-
- s := "Mysql://admin:password@serverhost.com:8081/server/page1?key=value&key2=value2#X"
-
- u, err := url.Parse(s)
- if err != nil {
- panic(err)
- }
- p(u.Scheme)
- p(u.User)
- p(u.User.Username())
- pass, _ := u.User.Password()
- p(pass)
- p(u.Host)
- host, port, _ := net.SplitHostPort(u.Host)
- p(host)
- p(port)
- p(u.Path)
- p(u.Fragment)
- p(u.RawQuery)
- m, _ := url.ParseQuery(u.RawQuery)
- p(m)
- p(m["key2"][0])
- }
Output:
mysql
admin:password
admin
password
serverhost.com:8081
serverhost.com
8081
/server/page1
X
key=value&key2=value2
map[key:[value] key2:[value2]]
value2
Go URL Parsing Example 2
- package main
-
- import (
- "io"
- "net/http"
- )
-
-
- func main() {
- http.HandleFunc("/company", func(res http.ResponseWriter, req *http.Request) {
- displayParameter(res, req)
- })
- println("Enter the url in browser: http://localhost:8080/company?name=Tom&age=27")
- http.ListenAndServe(":8080", nil)
- }
- func displayParameter(res http.ResponseWriter, req *http.Request) {
- io.WriteString(res, "name: "+req.FormValue("name"))
- io.WriteString(res, "\nage: "+req.FormValue("age"))
- }
Output:
Enter the url in browser: http://localhost:8080/company?name=Tom&age=27
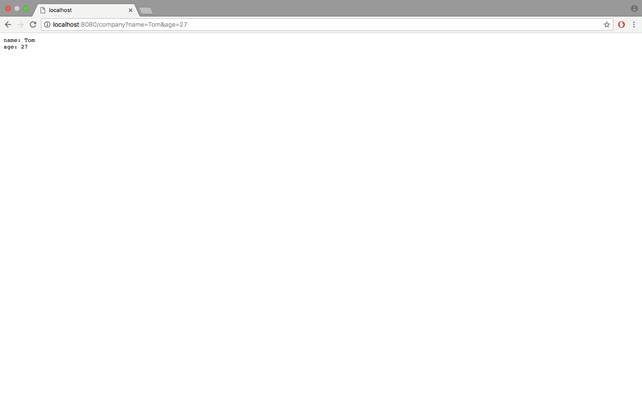
No comments:
Post a Comment