17. Explain Docker in .NET Core.
Docker is an open platform for developing, shipping, and running applications. It allows you to quickly isolate your applications from the infrastructure to transmit software. You should leverage this feature for managing infrastructure and deploying codes fast. It would help reduce the time needed between writing and running codes in infrastructure.
Three main functions:
- Quick and constant delivery of applications
- Responsive deployment and scaling
- Efficiently run more workloads on the same hardware
Take care following points while using Docker in .NET Core
- You can use the Docker client's CLI for managing images and containers
- You must adequately integrate Docker images, containers, and registries while designing and containerising applications or microservices
- Use Dockerfile for rebuilding images and distribute them with others
18. What is .NET Core CLI?
.NET Core CLI is part of .NET SDK that provides a cross-platform toolset to develop, create, and run .NET Core applications. You can install multiple versions of the toolset on your machine. You can use the following standard syntax to use CLI:
dotnet [verb] [arguments]
It provides four types of commands
- Basic commands: All commands required to develop applications like new, restore, build, run, etc.
- Project Modification commands: It allows you to use existing packages or add packages for developing applications.
- Advanced commands: It gives various commands to perform additional functions such as deleting nuget.
- Tool management commands: You can use these commands to manage tools.
19. What is Hosting Environment Management?
It is a new feature of .NET Core that permits you to work with multiple environments with no friction. You can use this feature through the available interface, Hosting Environment. The interface has been open since the first run of the application. Its execution depends on the environment variable and switch between the configuration files during runtime.
The interface reads a specific environment variable named "ASPNETCORE_ENVIRONMENT" and checks its value. Check its following values:
- If value: Development – You are running the application in Dev mode
- If value: Staging – You are running the application in staging mode
This feature permits you to manage the different environments as well.
20. Briefly explain Garbage Collection, its benefits, and its condition.
Garbage collection is another powerful feature of .NET Core. The primary function of this feature is to manage memory allocation and release. The .NET Core has "Zero Garbage Collector" to execute this function. You can call it Automatic Memory Manager.
Benefits:
- You don't need to put effort into releasing memory manually
- Efficient object allocation on the heap
- Ensure memory security by ensuring object's usage
- You can reclaim objects that are no longer needed, free the memory, and use it for other purposes.
Three conditions that allow garbage collection
- The system has low physical memory
- In case of an acceptable threshold
- When the GC method has been called
21. Discuss CTS types in .NET Core.
Common Type System or CTS standard defines and explains how to use data types in the .NET framework. The "System.Object" is the base type that derives other types in the singly rooted object hierarchy. It is a collection of data types, and Runtime uses it to implement cross-language integration.
You can categorise this into two types:
- Value types: This data type uses an object's actual value to represent any object. If you assign instance of value type to a variable, that variable is given a fresh copy of the value.
Examples: Built-in value types, User-defined value types, Enumeration, Structure
- Reference types: This data type uses a reference to the object's value to represent the objects. You can say it follows the concept of pointers. It doesn't create any copy if you assign a reference type to a variable that further points to original values.
Examples: Self-defining types like array, Pointer type, Interface Type
22. Explain CoreRT.
In .NET Core, CoreRT has been used as a native toolchain that performs compilation to translation. In other words, it compiles CIL byte code to machine code. The CoreRT uses ahead-of-complier, RyuJIT for compilation. You can also use it with other compilers to perform native compilation for UWP apps.
As a developer, you can utilise its following benefits:
- It is easy to work with one single file generated during compilation along with app, CoreRT, and managed dependencies.
- It works fast because of the prior execution of compiled code. You don't need to generate machine code or load the JIT compiler at runtime.
- Since it uses an optimised compiler, thus it generates faster output from higher quality code.
23. Why is Startup Class important?
The Startup is a critical class in the application. The following points make it imperative:
- It describes the pipeline of the web applications.
- You can use individual startups for each environment.
- It helps to perform the registration of all required middleware components.
- Reading and checking thousands of lines in different environments is tough, but you can use various startup classes to resolve it.
24. What do you mean by state management?
Regarding .NET Core frameworks, state management is a kind of state control object to control the states of the object during different processes. Since stateless protocol, HTTP has been used, which is unable to retain user values; thus, different methods have been used to store and preserve the user data between requests.
Approach Name | Storage Mechanism |
Cookies | HTTP Cookies, Server-side app code |
Session state | HTTP Cookies, Server-side app code |
Temp Data | HTTP Cookies, Session State |
Query Strings | HTTP Query Strings |
Hidden Fields | HTTP Form Fields |
HTTPContext.Items | Server-side app code |
Cache | Server-side app code |
25. What is the best way to manage errors in .NET Core?
There are mainly four ways to manage errors in .NET Core for web APIs.
- Developer Exception Page
- Exception Handler Page
- Exception Handle Lambda
- UseStatusCodePages
But, in all these four, the best way is "Developer Exception Page" as it provides detailed information (stacks, query string parameters, headers, cookies) about unhandled request exceptions. You can easily enable this page by running your applications in the development environment. This page runs early in the middleware pipeline, so you can easily catch the exception in middleware.
26. IS MEF still available in .NET Core?
Yes, MEF or Managed Extensibility Framework is still available. This library plays a major role in developing lightweight and extensible applications. You can easily use extensions without configuration. You can restore the extensions within and outside the application. You can smoothly perform code encapsulation and prevent fragile complex dependencies.
It has been considered outdated but is still available. If you want to use it, you must use it using some plugins systems and namespaces like "System.Composition", "System.ComponnetModel.Composition", and "Microsoft.Composition".
27. What is response caching in .NET Core?
During response caching, cache-related headers are mentioned in the HTTP responses of .NET Core MVC actions. Using these headers, we can specify how the client/proxy machine will cache responses to requests. This, in turn, reduces the number of client/proxy requests to the web server because the responses are sent from the cache itself.
As we can see in the below diagram, the first request has a complete cycle from client browser to proxy server and then subsequently to web server. Now, the proxy server has stored the response in the cache. For all the subsequent requests, the proxy server sends the response from the cache itself. Hence, the number of proxy/client requests to the web server is reduced.
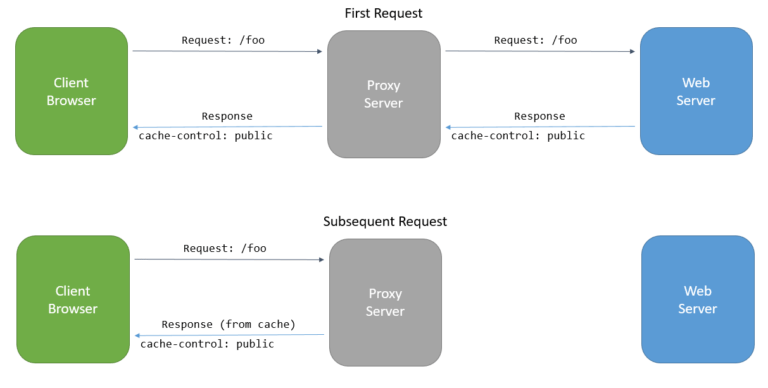
28. What is a generic host in .NET Core?
The generic host was previously present as ‘Web Host’, in .NET Core for web applications. Later, the ‘Web Host’ was deprecated and a generic host was introduced to cater to the web, Windows, Linux, and console applications.
Whenever a new application is started we are required to take care of the below points:
- Dependency Injection
- Configuration
- Logging
- Service lifetime management
.NET generic host called ‘HostBuilder’ helps us to manage all the above tasks since it is built on the original abstraction of these tools.
29. What is routing in .NET Core?
It is a process through which the incoming requests are mapped to the corresponding controllers and actions. The .NET Core MVC has a routing middleware to perform this task. This middleware matches the incoming HTTP requests to the executable request-handling code. We can define the routing in the middleware pipeline in the ‘Startup.Configure’ file.
As we can see in the below code snippet, there are two methods or pair of middleware to define routing:
- UseRouting: Adds route which matches the middleware pipeline.
- UseEndpoints: Adds end execution point to the middleware pipeline and runs the delegate of the endpoint.
30. What is Dependency Injection in .NET Core? Explain its advantages.
.NET Core has been designed to support Dependency Injection(DI), which means the application is loosely coupled. It is a technique to introduce Inversion Control(IoC) between the classes and their dependencies. In other words, the object maintains only that dependency which is required during that particular task. A dependency is an object on which another object depends, by dependency injection, the application becomes better testable, maintainable, and reusable.
Dependency Injection has three steps:
- An interface or base class is present to provide an abstraction for dependency implementation.
- Dependency is registered in a service container, a built-in container IServiceProvider is present in .NET Core.
- Service is injected into the constructor of the class where dependency is used.
Advantages of Dependency Injection:
- Code is flexible, implementation can be changed without much overhead.
- Code becomes easy to test because of the use of interfaces.
- Code is loosely coupled, clean, and easy to maintain.
No comments:
Post a Comment