1. What is .NET Core Framework, and how does it work?
.NET Core framework provides an open-source, accessible, and general-purpose platform to create and run applications onto different operating systems. The framework follows the object-oriented programming principles that we can use C#, .NET, VB, Perl, Cobol, etc., programming languages. The framework provides various built-in tools such as packages, classes, libraries, APIs, and other functionalities. We can create a diverse range of applications.
It works as follows:
- Once you have finished developing codes for required applications, you need to compile those application codes to Common Intermediate Language.
- The framework uses an assembly file to store the compiled code with an extension (.dll or .exe)
- Now, the Common Language Runtime (CLR) of the framework convert the compiled code to machine code (executable code) using the Just In Time (JIT) compiler.
- At last, we can execute this executable code on any specific architecture used by developers.
2. What is the latest version of .NET Core? Share one specific attribute.
The latest version of .NET Core is .NET Core 6.0, and its release date is July 12 2022, according to Microsoft Documentation. The newest release includes the .NET Runtime and ASP.NET Core Runtime. It has introduced Android, iOS, and macOS SDKs for developing native applications. You can check this documentation to know the setup instructions and develop .NET MAUI applications.
.NET Core has these 4 specific features:
- Cross-platform: It supports various platforms and is executable on windows, macOS, and Linux. You can easily port the codes from one platform to another platform.
- Flexibility: You can easily include codes in the desired app or install them per requirements. It means you can use one single consistent API model for all .NET applications with the help of the same library on various platforms.
- Open Source: You can use it by downloading it from the Github library. You don't need to pay to purchase a license. The framework has been licensed under MIT and Apache.
- Command-line tools: You can efficiently execute applications at the command line.
4. What is .NET Core used for?
You can use .NET Core in many ways:
- For developing and building web applications and services that run on diverse operating systems
- For creating Internet of Things applications and mobile backends
- For using any development tools on any operating system
- For creating and deploying applications to the cloud or other on-premises services.
- Flexibility, high performance, and lightweight features allow for the development of applications quickly in containers deployable on all operating systems.
5. Discuss critical components in .NET Core?
Since .NET Core is a modular platform thus, its components could be stacked into these three layers:
- A .Net runtime: It consists of different runtime libraries that allow you to perform functions such as type safety, load assemblies, garbage collections etc.
- A collection of Framework libraries: It also consists of libraries that offer utilities, primitive data types, etc.
- A collection of SDK tools and compilers: It permits you to work with .NET Core SDK quickly.
This stack could be divided into these four components:
Image Credit: .NET Core Components
6. What is the difference between .Net Core and Mono?
Point | .Net Core | Mono |
What is exactly? | A part of.NET framework which is specially optimised for designing modern apps and supporting developer workflows | It is also part of .NET family frameworks, but this framework is optimised for iOS, macOS, Android, and Windows devices by the Xamarin platform |
Best application Area |
|
|
Specific features |
|
|
App Models | UWP (Universal Windows Platform), ASP.NET Core | Xamarin iOS, Xamarin Android, Xamarin Forms, Xamarin Mac |
Base library | CoreFX Class Library | Mono Class Library |
7. What is .NET Core CoreFX?
CoreFX is the introductive class library for .NET Core. It consists of collection types, file systems, console, JSON, and XML for class library implementation. You can use this code as a single portable assembly. Since it provides platform-neutral code, thus you can share it across different platforms.
8. What is CoreCLR?
CoreCLR is the .NET execution engine in .NET Core. It consists of a garbage collector, JIT compiler, low-level classes, and primitive data types. Garbage collection and machine code compilation are its primary functions.
The following image shows .NET Core Compilation. You can clearly write codes in different languages that compliers like Roslyn would comply with. The compiler will generate the respective CIL code used by the JIT compiler for further compilation. Since CoreCLR is embedded in the JIT compiler, it would eventually generate machine code. Check its source code available on GitHub
Image Credit: dotnet-talk
9. How is .NET Core SDK different from .NET Core Runtime?
.NET Core SDK builds applications, whereas .NET Core Runtime runs the application. Consider SDK is a collection of all tools and libraries you need to develop .NET Core applications quickly like a compiler, CLI. Consider Runtime as a virtual machine consisting of runtimes libraries and helps you run those applications.
10. Where should you not use .NET Core?
Consider these application areas where you should prevent using .NET Core
- Avoid using current .NET framework applications in productions or migration because there is a possibility when you are unable to execute third libraries from apps running on the .NET core. Although, these libraries are executable from the .NET framework.
- Avoid using .NET Core in designing loosely coupled and new large monolithic applications. It is because of computability issues while consuming libraries with the .NET framework. You can create such applications by running on the top of the .NET framework and with the help of CLR libraries.
- Any applications that need sub frameworks like WPF, WebForms, Winforms as .NET Core don't support these.
- Prevent trying .NET Core in applications requiring higher level frameworks such as WCF, Entity Framework, and Windows Workflow Foundation.
11. What are the advantages of .NET Core?
- Cross-platform development and deployment: It can support application development on different platforms such as Windows, Linux, Mac, etc. Also, the deployment is supported on multiple platforms through containerization(Docker, Kubernetes, Service Fabric). This makes .NET completely portable and runnable on different platforms.
- Open-source: All .NET source code and documentation is freely available for download and contribution. This results in faster software releases, enormous support, and usage of the latest tools in development.
- Supports a plethora of applications: It has the capabilities to support a wide range of application types such as desktop, web, AI, cloud, mobile, IoT, gaming, etc.
- Secure: Provides easy-to-incorporate security measures like authentication, authorization, and data protection. It has mechanisms to protect the sensitive-data like keys, passwords, connection strings, etc. For e.g. in terms of authentication, ASP.NET Core Identity allows you to integrate your app with all major external providers.
- High performance: With every new release of the .NET core, the performance is improved for the benefit of users. For example, in .NET 5, the garbage collection is improved for faster speed, scalability, and reduction in memory resets’ cost. Detailed account of performance improvement in .NET 5.
- Flexible: Provides the flexibility to use any database and infrastructure as per choice. It provides the ability to change, evolve and grow easily according to external factors.
12. What is Kestrel?

Kestrel is an event-driven, I/O-based, open-source, cross-platform, and asynchronous server which hosts .NET applications. It is provided as a default server for .NET Core therefore, it is compatible with all the platforms and their versions which .NET Core supports.
Usually, it is used as an edge-server, which means it is the server which faces the internet and handles HTTP web requests from clients directly. It is a listening server with a command-line interface.
Advantages of Kestrel are:
- Lightweight and fast.
- Cross-platform and supports all versions of .NET Core.
- Supports HTTPS.
- Easy configuration
13. What do you know about .NET Core middleware?
Middleware is a layer, software, or simple class through which all the requests and responses have to go through. The middleware is assembled of many delegates in an application pipeline. Each component(delegate) in the pipeline of the middleware decides :
- To pass the request to the next component.
- Perform some processing on the request before or after passing it.
The below diagram shows a middleware request pipeline consisting of many delegates called one after another. Where black arrows mark the direction of execution. Each delegate in the diagram performs some operations before or after the next delegate.
More details from Microsoft’s documentation.

14. What are Razor Pages in .NET Core?
Razor Pages is a new server-side framework which works on a page-based approach to render applications in .NET Core. They are stored as a physical .cshtmlfile.
They have the HTML and code in a single file, without the need to maintain separate controllers, view models, action methods, etc. We can also have the code separate from the HTML in a different file which is attached to the Razor Page. Both types are shown below in the diagram:
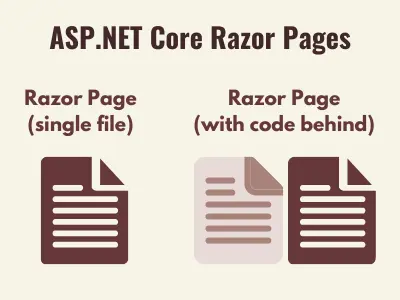
Razor Pages framework is flexible, lightweight, cohesive, page-based, easy to learn and maintain compared to MVC. It can be used in conjunction with traditional MVC (Model-View-Controller) architecture or Web-API controllers.
15. What are service lifetimes in .NET Core?
.NET Core supports a design pattern called ‘Dependency Injection’ which helps in the implementation of IoC(Inversion of Control). During registration, dependencies require their lifetime to be defined. The lifetime of service decides under what condition the instance of the service will be created and till what time it will be live.
There are three types of service lifetimes supported by .NET Core:
- Transient Service: Instance is created each time it is requested.
- Scoped Service: User-specific instance is created once per user and shared across all the requests.
- Singleton Service: Single Instance is created once a lifetime of the application.

16. What are the differences between .NET Core and .NET Framework?
.NET Core | .NET Framework |
Completely open-source. | Few components are open-source. |
Compatible with Linux, Windows, and Mac operating systems. | Compatible with only Windows. |
Does not support desktop application development. | Supports web and desktop application development. |
Supports microservices development. | Does not support microservices development. |
Lightweight for Command Line Interface(CLI). | Heavy for Command Line Interface. |
No comments:
Post a Comment