In this article I will explain with an example, how to export Crystal Report to Word, Excel, PDF and CSV in Windows Forms (WinForms) Application using C# and VB.Net.
The Crystal Report will be populated using Typed DataSet in Windows Forms (WinForms) Application using C# and VB.Net.
Database
Here I am making use of Microsoft’s Northwind Database. The download and install instructions are provided in the following article.
Configuring Crystal Report in Windows Forms Application
The Crystal Report configuration has been covered in the following article Basic Crystal Report Tutorial with example in Windows Forms (WinForms) Application using C# and VB.Net.
Form Design
The Form consists of a CrystalReportViewer control, four RadioButtons in GroupBox and a Button.
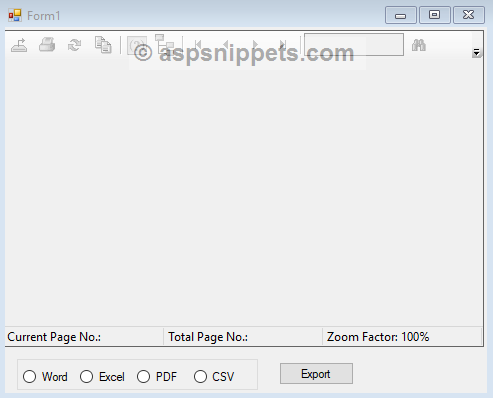
Namespaces
You will need to import the following namespaces.
C#
using System.Data;
using System.Data.SqlClient;
using CrystalDecisions.Shared;
using CrystalDecisions.CrystalReports.Engine;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports CrystalDecisions.Shared
Imports CrystalDecisions.CrystalReports.Engine
Populating the Crystal Report from Database
Inside the Form Load event, first the Customers DataSet is populated with records from the Customers Table.
The Customers DataSet is set as a DataSource for the Crystal Report.
Finally, the Crystal Report is set as ReportSource for the CrystalReportViewer control.
C#
private void Form1_Load(object sender, EventArgs e)
{
CustomerReport crystalReport = new CustomerReport();
Customers dsCustomers = GetData();
crystalReport.SetDataSource(dsCustomers);
this.crystalReportViewer1.ReportSource = crystalReport;
this.crystalReportViewer1.RefreshReport();
}
private Customers GetData()
{
string constr = @"Data Source=.\Sql2005;Initial Catalog=Northwind;Integrated Security = true";
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand("SELECT TOP 20 * FROM Customers"))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
sda.SelectCommand = cmd;
using (Customers dsCustomers = new Customers())
{
sda.Fill(dsCustomers, "DataTable1");
return dsCustomers;
}
}
}
}
}
VB.Net
Private Sub Form1_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
Dim crystalReport As New CustomerReport()
Dim dsCustomers As Customers = GetData()
crystalReport.SetDataSource(dsCustomers)
Me.crystalReportViewer1.ReportSource = crystalReport
Me.crystalReportViewer1.RefreshReport()
End Sub
Private Function GetData() As Customers
Dim constr As String = "Data Source=.\Sql2005;Initial Catalog=Northwind;Integrated Security = true"
Using con As New SqlConnection(constr)
Using cmd As New SqlCommand("SELECT TOP 20 * FROM Customers")
Using sda As New SqlDataAdapter()
cmd.Connection = con
sda.SelectCommand = cmd
Using dsCustomers As New Customers()
sda.Fill(dsCustomers, "DataTable1")
Return dsCustomers
End Using
End Using
End Using
End Using
End Function
Exporting the Crystal Report to Word, Excel, PDF or CSV file
When the Export Button is clicked, based on RadioButton selection the Crystal Report is exported to the desired format i.e. Word, Excel, PDF or CSV file.
C#
private void btnExport_Click(object sender, EventArgs e)
{
if (rbWord.Checked)
{
crystalReport.ExportToDisk(ExportFormatType.WordForWindows, @"D:\Files\CustomerReport.doc");
}
else if (rbExcel.Checked)
{
crystalReport.ExportToDisk(ExportFormatType.Excel, @"D:\Files\CustomerReport.xls");
}
else if (rbPDF.Checked)
{
crystalReport.ExportToDisk(ExportFormatType.PortableDocFormat, @"D:\Files\CustomerReport.pdf");
}
else if (rbCSV.Checked)
{
crystalReport.ExportToDisk(ExportFormatType.CharacterSeparatedValues, @"D:\Files\CustomerReport.csv");
}
}
VB.Net
Private Sub btnExport_Click(sender As Object, e As EventArgs) Handles btnExport.Click
If rbWord.Checked Then
crystalReport.ExportToDisk(ExportFormatType.WordForWindows, "D:\Files\CustomerReport.doc")
ElseIf rbExcel.Checked Then
crystalReport.ExportToDisk(ExportFormatType.Excel, "D:\Files\CustomerReport.xls")
ElseIf rbPDF.Checked Then
crystalReport.ExportToDisk(ExportFormatType.PortableDocFormat, "D:\Files\CustomerReport.pdf")
ElseIf rbCSV.Checked Then
crystalReport.ExportToDisk(ExportFormatType.CharacterSeparatedValues, "D:\Files\CustomerReport.csv")
End If
End Sub
Screenshots
The Crystal Report
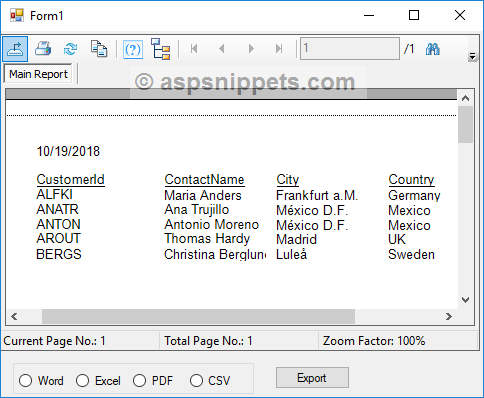
Crystal Report exported to Word Document
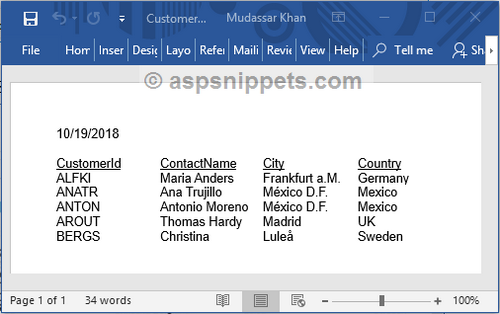
Crystal Report exported to Excel Spreadsheet
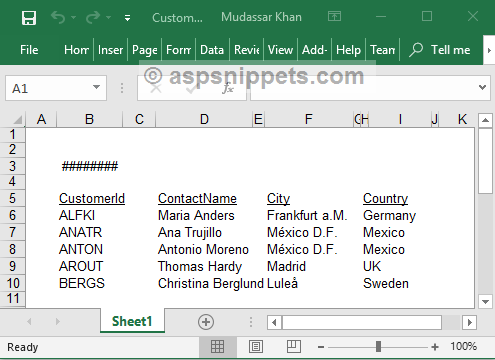
Crystal Report exported to PDF file
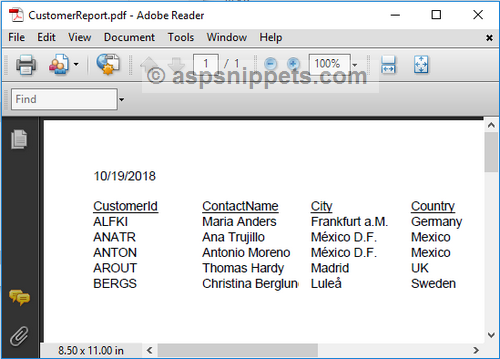
Crystal Report exported to CSV file
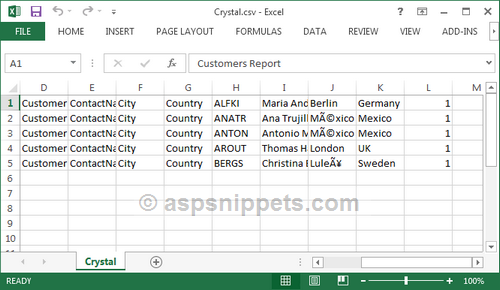
No comments:
Post a Comment